ShowTable of Contents
The Clipboard API offers a simple way to write various data formats to the system clipboard. This article demonstrates some of the available methods and their syntax.
For a complete API reference, please refer to the
XPages2Eclipse Base API Javadocs.
Access to API
You can get access to this API by getting a new instance of
IRemoteClipboardAccess
from the
ClipboardAPI
factory class:
var conn=X2E.createConnection();
var clipboardAccess=com.x2e.ClipboardAPI.getClipboardAccess(conn);
This instance has methods to write plain text, a file and an image to the clipboard:
clipboardAccess.setTextContent("Hello world!");
clipboardAccess.setHTMLContent("<b>Hello world!</b>");
clipboardAccess.setFileContent("c:\temp\archiv.zip");
clipboardAccess.setImageContent("c:\temp\image.png");
Hint:
XPages2Eclipse uses the Eclipse class
org.eclipse.swt.graphics.Image
internally to load the image. This class supports the file formats BMP, ICO, JPEG, GIF, png and TIFF.
Sample application
Our sample application lets you enter text or select files and images which can then be copied to the clipboard with a button click.
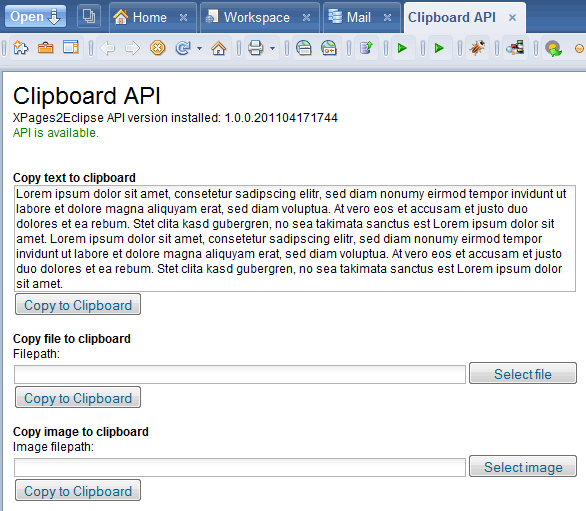
The file and image selection actions are leveraging the
IRemoteFileDialogTools
that are available from the
PlatformUI API class.
To select a file, we are using a pretty simple syntax, because any filetype can be selected:
var conn=X2E.createConnection();
var platformUI=com.x2e.PlatformUIAPI.getUI(conn);
var fdTools=platformUI.getFileDialogTools();
var selPath=fdTools.showFileOpenDialog();
if (selPath) {
getComponent("filePath").setValue(selPath);
}
The image selection uses a more advanced and powerful call syntax:
var conn=X2E.createConnection();
var platformUI=com.x2e.PlatformUIAPI.getUI(conn);
var fdTools=platformUI.getFileDialogTools();
var filterNames=["All images", "All files"];
var filterExt=["*.jpg;*.jpeg;*.png;*.gif", "*.*"];
var filterIndex=0;
var filterPath=null;
var fileName=null;
var overwrite=false;
var dlgText=null;
//use bitmask OPEN+SINGLE (4096+4) for selection type
var selPath=fdTools.showFileDialog(4096+4, filterNames, filterExt, filterIndex,
filterPath, fileName, overwrite, dlgText);
if (selPath && selPath.length>0) {
//method returns an array of paths even for single selection
getComponent("imagePath").setValue(selPath[0]);
}
These code snippets are executed in the onClick events of the "select file" and "select image" buttons to display file dialogs from server side JavaScript code, write the path of the selected files in a UI field and do a partial or full refresh of the XPage to display the path to the user.
Please note that the sample code increases the
submit latency value to avoid that the XPages browser code displays timeout error dialogs when the file selection dialog is opened for a long amount of time.
The sample application can be downloaded
here.